HTML image maps are a powerful tool in web design, allowing you to make different parts of an image clickable and perform various actions. Understanding how to track click events on these maps can provide valuable insights into user interaction and enhance the functionality of your website.
For a deeper understanding of html image maps, read our comprehensive article on it here.
Understanding Click Events
Click events capture interactions between the user and the site, providing valuable insights into how users engage with your content. In JavaScript, the addEventListener
method is a powerful tool that attaches an event listener to an element. This method allows you to specify the type of event to listen for (e.g., 'click') and a function to execute when the event occurs.
Here's a brief example of how it works:
document.getElementById('yourElementId').addEventListener('click', function() {
alert('Element clicked!');
});
This method enhances the interactivity of your site by enabling dynamic responses to user actions, such as clicks on an HTML image map.
Besides the addEventListener
method, the onclick
attribute offers a straightforward alternative for attaching click events directly within HTML elements. By adding the onclick
attribute to an element, you can define a JavaScript function that executes when the element is clicked.
This approach simplifies event handling, especially for basic interactions, making it accessible for those with limited JavaScript knowledge. In our example below, we are using this.
Basic Setup for Tracking Clicks on Image Maps
To begin tracking clicks on an HTML image map, you first need to understand the basics of the <map>
and <area>
tags. Adding an onclick
attribute to your <area>
elements is the simplest way to start capturing click events.
Adding the onclick
Listener
Including an onclick
listener in the <area>
element of your image map allows you to call a JavaScript function whenever that area is clicked. Here’s how you can add this attribute:
<map name="workmap" id="clickableimagearea">
<area shape="poly" coords="123, 456, 789" onclick="trackClick('counted');">
</map>
Creating the Tracking Function in JavaScript
The trackClick
function defined in the onclick
attribute is responsible for logging the click event. Here's a simple implementation:
<script type="text/javascript">
var count = 0;
function trackClick(id) {
count++;
document.getElementById(id).innerHTML = "This has been clicked " + count + " times.";
}
</script>
Demo
We have a demo below to showcase this effect
Try hovering around the left eye and right eye of the image of the puppy below. Then go ahead to click them to see the labels below the image change according to how many times you clicked the area around the eyes.
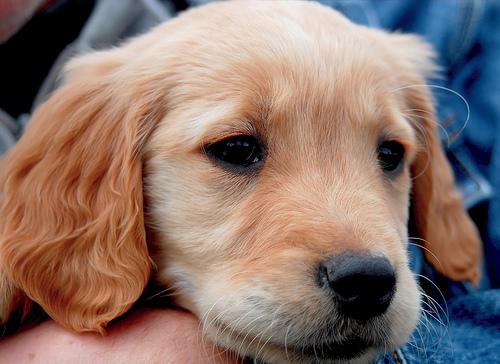
The left eye has been clicked 0 times.
The right eye has been clicked 0 times.
And here is the exact code for it:
<map name="workmap" id="clickableimagearea">
<area
shape="poly"
coords="161.298939617426,89.19757029535081,313.678122205317,102.5772058396534,282.4589726019442,195.4913415639772,176.1652013333178,192.5180892207988"
onclick="trackClick(event, 'left');"
style="cursor: pointer;"
>
<area
shape="poly"
coords="363.4800989535545,120.4167198987236,427.4050243318893,113.7269021265722,428.8916505034785,197.7212808213609,344.8972718086898,205.8977247651014"
onclick="trackClick(event, 'right');"
style="cursor: pointer;"
>
</map>
<script src="https://cdn.jsdelivr.net/npm/jquery@3.7.1/dist/jquery.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/image-map-resizer@1.0.10/js/imageMapResizer.min.js"></script>
<script>
$('map#clickableimagearea').imageMapResize();
</script>
<script type="text/javascript">
var count = 0;
function trackClick(event, id) {
event.preventDefault();
event.stopPropagation();
count++;
document.getElementById(id).innerHTML = `The <strong>${id}</strong> eye has been clicked <strong>${count}</strong> times.`;
}
</script>
Utilizing Our Tool
To streamline the process of creating responsive and interactive image maps, consider using our free online HTML image map generator tool. It saves you time and effort, making it easier to implement sophisticated features like click event tracking.
Testing and Debugging
You may encounter issues like the click event not firing or the counter not updating. These can often be resolved by ensuring the id
referenced in your JavaScript matches the id
of your elements and verifying the script is loaded correctly in your HTML.
Integration with Analytics
For a deeper analysis, consider integrating click event tracking with web analytics tools. This can provide insights into user behavior and help refine your content strategy.
If you are using Google Analytics via Google tag Manager, you can easily setup Google Tag Manager to listen for the click events on your image map.